ES6
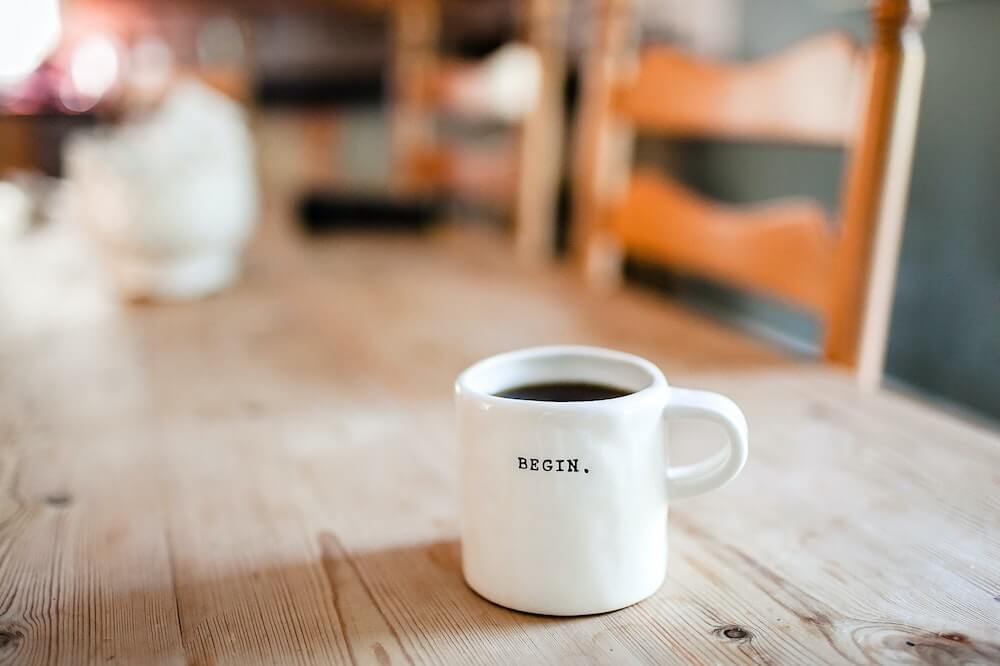
短篇 React 學習筆記。
ES6 for React
JavaScript = a “dialect” of ECMAScript
ES6 = ECMAScript 2015 = JavaScript standard.
React uses ES6. ES6 is also getting popularity for writing server applications and services via Node.js.
Variables
In ES6, variables are declared using const, let and var keywords.
We cannot redeclare const or let variables.
And, we can only reassign let variable.
Block Scope vs Function Scope
var is function scoped. It can be accessed everywhere.
let is a block scoped. It can only be accessed inside the block where it is defined.
New Update: Arrow Function & Regular Function
Regular Function
1
2
3
var hello = function(name) {
return name
}
ES6 Arrow function
1
let hello = (name) => { return name }
Even more ways to define arrow functions…
We can omit {}, if there’s only one expression
1
let hello = (num1, num2) => num1 + num2;
We can omit (), if there’s only one argument
1
let hello = name => name;
We can use _, if there’s no argument
1
let hello = _ => 1 + 2;
this keyword
In regular function, the this keyword represents the object called inside a function.
In arrow function, the this keyword represents the object that defined the function, which is the window. object.
Template Literals
Quoted between ``, string literals that can include expressions. Like this…
1
2
let name = 'Boop';
let greeting = `Hello, ${name}`;
Destructuring: Arrays
1
2
let array = [1,2,3,4,5];
let [value1,value2,value3] = array;
this is equivalent to
1
2
3
4
let array = [1,2,3,4,5];
let value1 = array[0];
let value2 = array[1];
let value3 = array[2];
Destructuring: Objects
Similar to destructuring array…
1
2
3
4
5
6
let obj = {
key1: 'boop',
key2: 'foo',
key3: 'bar'
};
let [key1,key2,key3] = obj;
Spread Operator
The spread operator spreads the values in an iterable, such as array & strings, across arguments or elements.
1
2
3
4
5
6
let fruits = ['apples','bananas','strawberry'];
let veggies = ['cabbage','asparagus','green onions'];
let grocery = [...fruits,...veggies];
console.log(grocery);
// ['apples','bananas','strawberry','cabbage','asparagus','green onions']
Spread operator can also be used to clone an array & objects in an arrow function.
1
2
3
4
5
6
const dog1 = {
name: 'Boop',
breed: 'Corgi'
}
const dog2 = {...dog1}
Rest Parameter
This is used to pass an arbitrary number of argument, and to process these args within the arrow function.
Rest parameters are array instances.
We use rest parameters if we need extra parameters other than the specified ones.
1
2
3
4
5
6
7
let foo = function(a, b, ...args) {
console.log(args);
}
foo(1,2); // [], nothing will print inside the array, because we don't have extra parameters
foo(1,2,3,4,5) // [3,4,5]
Difference between rest parameter and normal arguments
Rest parameters returns an array by default, so methods like map, sort, shift, forEach… can be used directly. But for normal args, they need to be converted into an array because normal args are objects.