Virtual DOM & DOM Manipulation
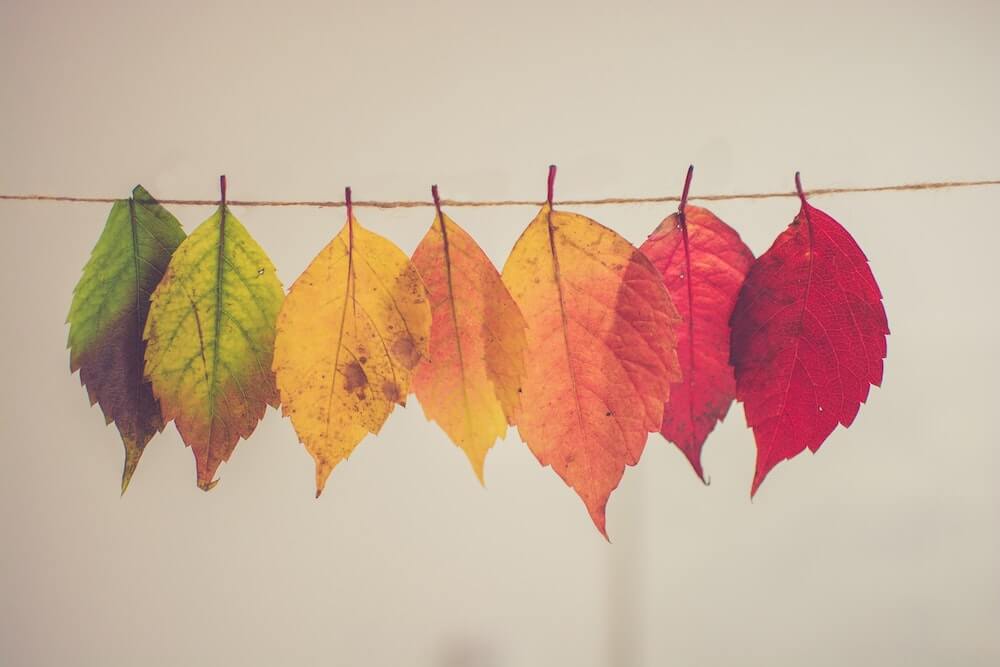
短篇 React 學習筆記。
Virtual DOM
React creates a tree of custom objects, each representing a part of the DOM.
If we create a p element inside a div, React will create React.div object and React.p object.
React will then modify these objects quickly when needed (modifying only what’s required) rather than changing the real DOM.
Before it renders the component, React will determine what needs to be changed using the virtual DOM. Then React will get virtual DOM and real DOM in sync.
Virtual DOM = blueprint
Virtual DOM contains the required details needed to construct the DOM. But it doesn’t require all the heavy parts of the real DOM. Thus, making virtual DOM creation much faster than actual DOM.
DOM Manipulation
DOM Manipulation = dynamically changing the content of web page.
Reconciliation = changes made to the virtual DOM and then sync to the real DOM.
Diffing = process when React figures out which objects has been changed
Process
- React updates virtual DOM
- States of virtual DOM will be compared (previous + updated), to identify what has been changed. This is done via Diffing Algorithm.
- Changed objects will get updated to the real DOM
Example of Diffing Algorithm
When root is changed, React will delete the tree and rebuild the entire tree.
1
2
3
4
5
// old
<div><App /></div>
// new
<span><App /></span>
When attribute is changed, React will only change the attribute.
1
2
3
4
5
// old
<div id="root"></div>
// new
<div id="boop"></div>
When new child element is added at the end of a list, React will simply add it to the list.
1
2
3
4
5
6
7
8
9
10
11
12
// old
<ul>
<li>hi</li>
<li>hi1</li>
</ul>
// new
<ul>
<li>hi</li>
<li>hi1</li>
<li>hi2</li>
</ul>