Functional Components vs Class Components
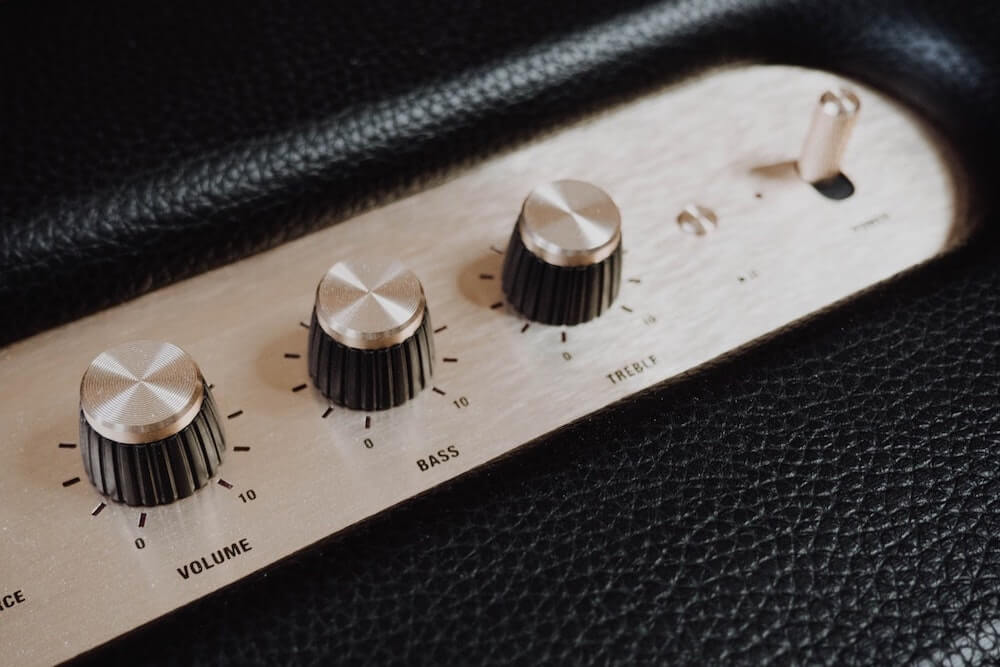
短篇 React 學習筆記。
About Components
Core building blocks of React.
Multiple individual components forms a parent component.
2 types of components in React:
- Functional
- Class
Before React Hooks were introduced, functional components were generally used for style (aka the dumb/stateless/presentational component) and class components were used for logic (smart component/stateful/container components). This practice has since been deprecated.
Functional Components
After React Hooks are introduced, functional components are used to store state using react hooks.
Like the name implies, think of functional component as a function.
Example:
1
2
3
function Welcome(props) {
return <h1>Hello, {props.name} </h1>;
}
1
const element = <Welcome name="Boop" />
Props should never be modified!
When should you use functional components?
For React < 16.8…
- When your comp. simply receive props, doesn’t have a state and renders smth (dumb/stateless/presentational comp.)
For React > 16.8… (React Hooks were introduced)
- Func. comp. can now have its own state via React Hooks
- Preferred over class comp. bc of React Hooks now.
Class Components
Class components were preferred before React Hooks were introduced.
Basically ES6 classes.
Similar to functional component, class comp. optionally receives props as input and renders JSX.
Class comp. can also maintain private internal state of a particular comp.
Example:
1
2
3
4
5
class Welcome extends React.Component {
render(){
return <h1>Hello, {this.props.name} </h1>;
}
}
Comp. should always start with capital letter.
Again, props should never be modified!
Drawbacks
- Class comp. is slower than fun. comp in terms of performance. This difference increases as the number of comp in the app increases.
- Slightly inefficient to use bc it requires more coding.