State
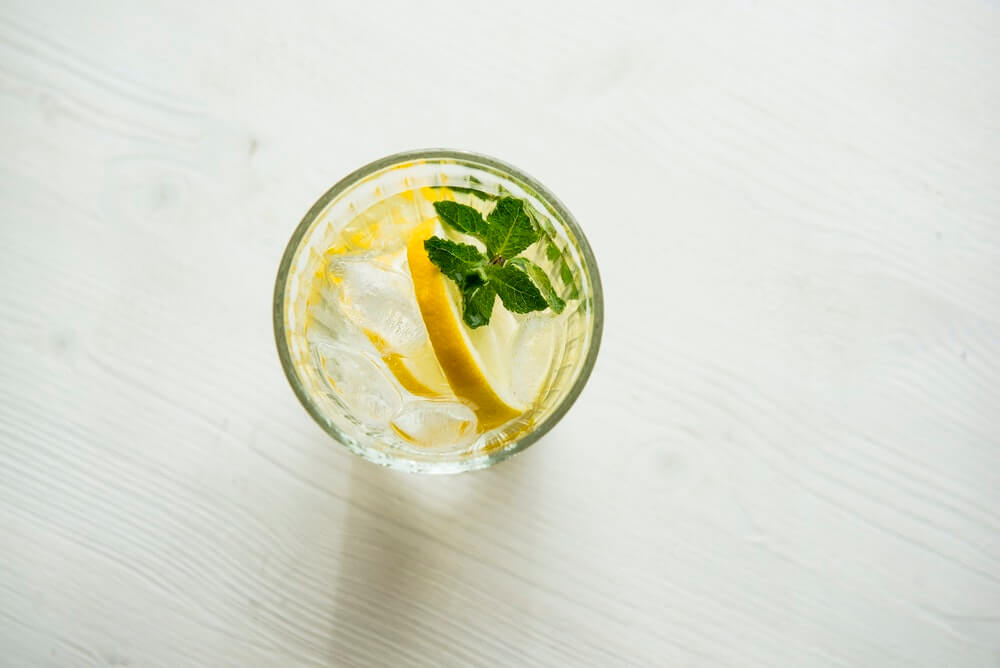
短篇 React 學習筆記。
State
What is State
Object that holds information that might change over the lifetime of the component.
Used to track data from form input, dynamic data and API.
Example - Local State:
Assignment:
1
2
3
4
5
6
7
8
class Welcome extends React.component {
constructor(props){
super(props);
this.state = {
name: 'Boop';
}
}
}
We assign the initial state in the constructor.
Reading State:
1
console.log(this.state.name);
Updating State:
1
2
3
4
5
// wrong
this.state.name = 'Foo';
// correct
this.setState({name: 'Foo'});
We need to replace the entire state object.
Updating State
State updates may be asynchronous and are merged.
Take a look:
1
2
3
4
5
6
this.state = {
count: 0;
}
this.setState({count: count + 1}); // count: 1
this.setState({count: count + 1}); // count: 1
It should give you 2 right? No
Both the setState count here is enqueued when its value is 0.
So what do we do if we want to access previous state?
We pass a function in setState that takes the previous state and updates it in a synchronous manner.
Like this:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
this.state = {
count: 0;
}
this.setState(
(prevState) => {
return {
count: prevState.count + 1
}
}
);
// count: 1
this.setState(
(prevState) => {
return {
count: prevState.count + 1
}
}
);
// count: 2
useState Hooks (React 16.8)
**To be further discussed in details in a separate post
Example code with functional component:
1
2
3
4
5
6
7
8
9
10
11
12
13
import React, { useState } from 'react';
export default function Welcome () {
const [count, useCount] = useState(0);
return (
<div>
Counter: { count } <br />
<button onClick={()=> useCount( count + 1 )}>
Add Count</button>
</div>
);
}
Note: Hooks aren’t supported in class comp.