useEffect Hooks
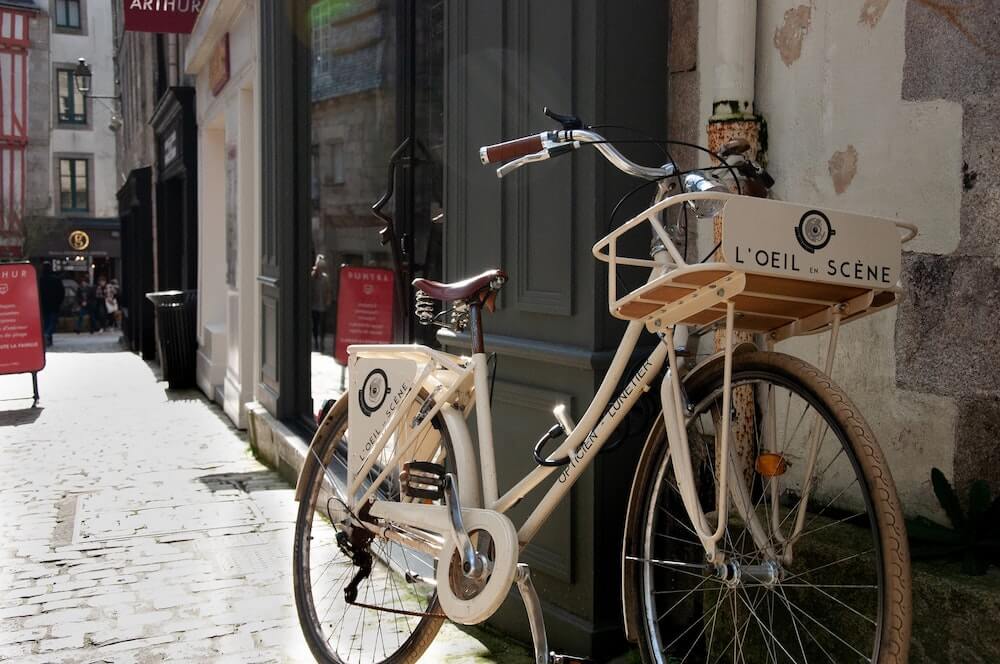
短篇 React 學習筆記。
useEffect Hooks
Use this Hook to tell React what to do when the component needs to perform a task after rendering.
Sample:
This side effect will execute on every render including when the component has mounted and every time it updates.
Let’s use fetch user from an API as an example…
componentDidMount()
1
2
3
4
5
6
7
8
useEffect(() => {
// componentDidMount code goes here
fetchUserApi(id).then((user) => {
setUser(user)
})
}, []);
^Bc we passed an empty array as the second parameter, the useEffect hook will only execute once.
componentDidUpdate(prevProps)
1
2
3
4
5
6
7
useEffect(() => {
// ComponentDidUpdate code goes here
fetchUserApi(id).then((user) => {
setUser(user)
})
}, [id]);
^This effect will execute only once, when component is updated (includes re-rendering).
componentWillUnmount()
1
2
3
4
5
6
useEffect(() => {
return() => {
// componentWillUnmount code goes here
setUser(null)
}
}, []);
All Three Combined
1
2
3
4
5
6
7
8
9
10
useEffect(() => {
fetchUserApi(id).then((user) => {
setUser(user)
})
return() => {
setUser(null)
}
}, [id]);