Event Handling
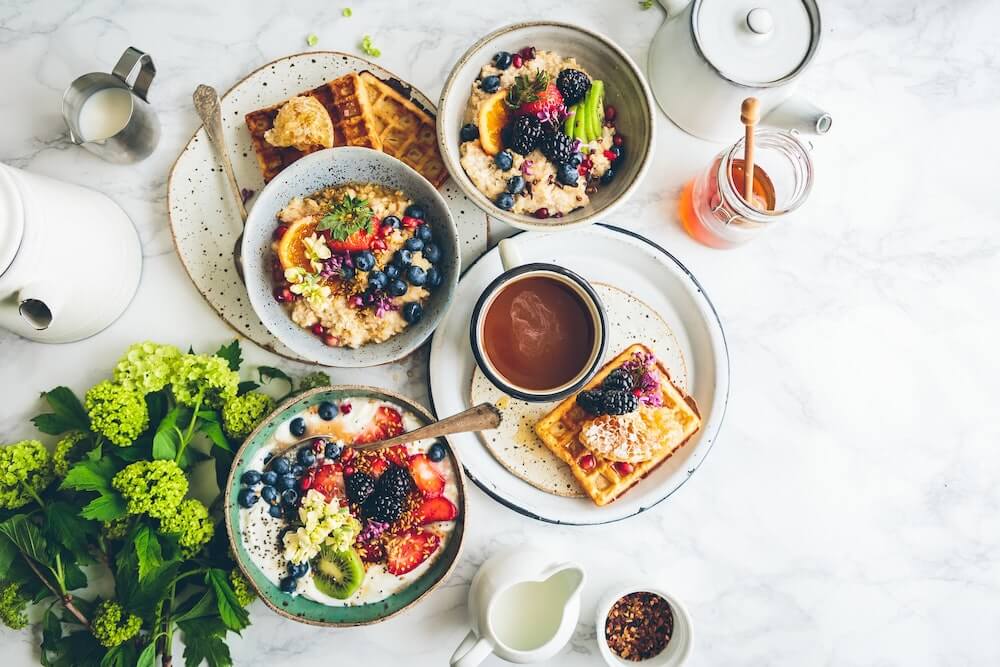
短篇 React 學習筆記。
Handling Events
Events are fired when user interacts with the application.
Some examples in React are…
- onclick
- onchange
- onfocus
- onblur
Handling events in React is very similar to handling events in DOM elements.
But there are still some differences…
Example of event handling in functional component
1
2
3
4
5
6
7
8
9
10
11
function FunctionEventHandling() {
function handleClick(e){
console.log('I am eating!!');
}
return (
<div>
<button onClick={handleClick}>Let's Eat!</button>
</div>
);
}
But why is it this:
1
<button onClick={handleClick}>Let's Eat!</button>
and not this?
1
<button onClick={handleClick()}>Let's Eat!</button>
Bc the event handling function should NOT be called with ().
It we call the handler with (), the function will be executed before the button is clicked!!
Event handler is a function not a function call!
Example of event handling in class component
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
class EatingToggle extends React.Component {
constructor(props){
super(props);
this.state = {
isEating: true
};
this.handleClick = this.handleClick.bind(this);
}
handleClick(){
this.setState( state => {
isEating: !state.isEating
})
}
render(){
return (
<div>
<button onClick={this.handleClick}>
{this.state.isEating ? 'I am eating lah!' : 'Nope, not eating'}
</button>
</div>
);
}
}
`